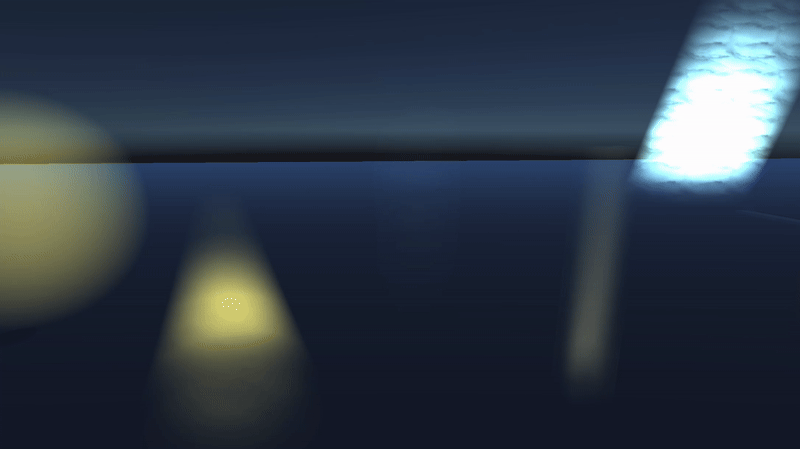
As of version 1.6.0 we’ve made it much easier to animate your lights at runtime. Whenever you have a reference to your VolumetricLight
you can adjust the settings of this light using the settings
field. Note that these changes don’t persist after play mode ends, so you can go wild! You can animate LightProfiles in the same way to animate multiple lights at the same time.
Directly setting the values
Here’s a simple script showing how to animate light properties manually by setting the value every frame:
using Sparrow.VolumetricLight;
using UnityEngine;
public class SimpleAnimationExample : MonoBehaviour
{
[SerializeField] VolumetricLight m_Light = default;
void Update()
{
m_Light.settings.intensityMultiplier = (Mathf.Sin(Time.time) + 1);
}
}
Using DOTween
You can also use a package like DOTween to animate lights. Here’s a simple example of how it works:
using Sparrow.VolumetricLight;
using UnityEngine;
using DG.Tweening;
public class SimpleAnimationExample : MonoBehaviour
{
[SerializeField] VolumetricLight m_Light = default;
private void Start()
{
DOTween.To(x => m_Light.settings.intensityMultiplier = x, 0, 3, 20f);
}
}